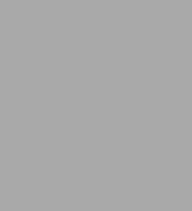
The Art of WebAssembly: Build Secure, Portable, High-Performance Applications
304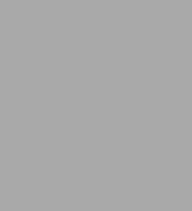
The Art of WebAssembly: Build Secure, Portable, High-Performance Applications
304Paperback
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
Related collections and offers
Overview
WebAssembly is the fast, compact, portable technology that optimizes the performance of resource-intensive web applications and programs. The Art of WebAssembly is designed to give web developers a solid understanding of how it works, when to use it (and when not to), and how to develop and deploy WebAssembly apps.
First you’ll learn how to optimize and compile low-level code, debug and evaluate WebAssembly, and represent WebAssembly in the human-readable WebAssembly Text (WAT) format. Once you have the basics down, you’ll build a browser-based collision detection program, work with browser rendering technologies to create graphics and animations, and see how WebAssembly interacts with other web languages.
You’ll also learn how to:
The Art of WebAssembly will help you make sense of this powerful technology to boost the performance of your web applications.
Product Details
ISBN-13: | 9781718501447 |
---|---|
Publisher: | No Starch Press |
Publication date: | 05/25/2021 |
Pages: | 304 |
Sales rank: | 1,012,929 |
Product dimensions: | 6.90(w) x 9.10(h) x 0.90(d) |
About the Author
Table of Contents
Foreword xv
Acknowledgments xvii
Introduction xix
Who Should Read This Book xix
Why Users Are Interested in WebAssembly xx
Why the World Needs WebAssembly xxi
What's in This Book xxi
1 An Introduction To Webassembly 1
What Is WebAssembly? 2
Reasons to Use WebAssembly 3
Better Performance 3
Integrating Legacy Libraries 4
Portability and Security 4
JavaScript Skeptics 4
WebAssembly's Relationship with JavaScript 5
Why Learn WAT? 6
WAT Coding Styles 7
The Embedding Environment 11
The Browser 11
WASI 11
Visual Studio Code 12
Node. js 12
Our First Node.js WebAssembly App 14
Calling the WebAssembly Module from Node.js 15
The then Syntax 16
The Time Is Now 17
2 Webassembly Text Basics 19
Writing the Simplest Module 20
Hello World in WebAssembly 20
Creating Our WAT Module 21
Creating the JavaScript File 23
WAT Variables 25
Global Variables and Type Conversion 25
Local Variables 29
Unpacking S-Expressions 30
Indexed Variables 32
Converting Between Types 32
If/else Conditional Logic 34
Loops and Blocks 37
The block Statement 37
The loop Expression 38
Using block and loop Together 39
Branching with br_table 42
Summary 43
3 Functions and Tables 45
When to Call Functions from WAT 46
Writing an is_prime Function 46
Passing Parameters 46
Creating Internal Functions 47
Adding the is-prime Function 49
The JavaScript 52
Declaring an Imported Function 54
JavaScript Numbers 55
Passing Data Types 55
Objects in WAT 55
Performance Implications of External Function Calls 55
Function Tables 59
Creating a Function Table in WAT 59
Summary 67
4 Low-Level Bit Manipulation 69
Binary, Decimal, and Hexadecimal 70
Integers and Floating-Point Arithmetic 71
Integers 71
Floating-Point Numbers 73
High-and Low-Order Bits 77
Bit Operations 78
Shifting and Rotating Bits 79
Masking Bits with AND and OR 80
XOR Bit Flip 83
Big-Endian vs. Little-Endian 84
Summary 85
5 Strings In Webassembly 87
ASCII and Unicode 88
Strings in Linear Memory 88
Passing the String Length to JavaScript 88
Null-Terminated Strings 90
Length-Prefixed Strings 93
Copying Strings 95
Creating Number Strings 101
Setting a Hexadecimal String 105
Setting a Binary String 110
Summary 113
6 Linear Memory 115
Linear Memory in WebAssembly 116
Pages 117
Pointers 119
JavaScript Memory Object 121
Creating the WebAssembly Memory Object 121
Logging to the Console with Colors 122
Creating the JavaScript in store_data.js 123
Collision Detection 125
Base Address, Stride, and Offset 126
Loading Data Structures from JavaScript 127
Displaying the Results 129
Collision Detection Function 130
Summary 138
7 Web Applications 139
The DOM 140
Setting Up a Simple Node Server 140
Our First WebAssembly Web Application 142
Defining the HTML Header 143
The JavaScript 143
The HTML Body 145
Our Completed Web App 146
Hex and Binary Strings 147
The HTML 147
The WAT 150
Compile and Run 154
Summary 156
8 Working With the Canvas 157
Rendering to the Canvas 158
Defining the Canvas in HTML 158
Defining JavaScript Constants in HTML 159
Creating Random Objects 161
Bitmap Image Data 162
The requestAnimationFrame Function 163
The WAT Module 164
Imported Values 164
Clearing the Canvas 165
Absolute Value Function 166
Setting a Pixel Color 167
Drawing the Object 169
Setting and Getting Object Attributes 171
The $main Function 173
Compiling and Running the App 183
Summary 184
9 Optimizing Performance 185
Using a Profiler 186
Chrome Profiler 186
Firefox Profiler 192
Wasm-opt 196
Installing Binaryen 196
Running wasm-opt 196
Looking at Optimized WAT Code 198
Strategies for improving Performance 199
Inlining Functions 199
Multiply and Divide vs. Shift 202
DCE 204
Comparing the Collision Detection App with JavaScript 205
Hand Optimizing WAT 208
Logging Performance 209
More Sophisticated Testing with benchmark.js 213
Comparing WebAssembly and JavaScript with -print-bytecode 219
Summary 222
10 Debugging Webassembly 223
Debugging from the Console 224
Logging Messages to the Console 229
Using Alerts 232
Stack Trace 233
The Firefox Debugger 238
The Chrome Debugger 243
Summary 245
11 Assemblyscript 247
Assembly Script CLI 248
Hello World AssemblyScript 249
JavaScript for Our Hello World App 251
Hello World with the AssemblyScript Loader 253
AssemblyScript String Concatenation 254
Object Oriented Programming in AssemblyScript 256
Using Private Attributes 258
JavaScript Embedding Environment 259
AssemblyScript Loader 260
Extending Classes in AssemblyScript 263
Performance of Loader vs. Direct WebAssembly Calls 264
Summary 267
Final Thoughts 269
Index 271