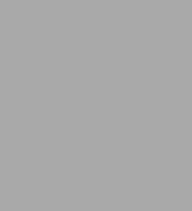
Spring Microservices in Action, Second Edition
448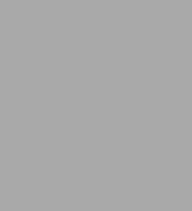
Spring Microservices in Action, Second Edition
448eBook
Available on Compatible NOOK devices, the free NOOK App and in My Digital Library.
Related collections and offers
Overview
Summary
By dividing large applications into separate self-contained units, Microservices are a great step toward reducing complexity and increasing flexibility. Spring Microservices in Action, Second Edition teaches you how to build microservice-based applications using Java and the Spring platform. This second edition is fully updated for the latest version of Spring, with expanded coverage of API routing with Spring Cloud Gateway, logging with the ELK stack, metrics with Prometheus and Grafana, security with the Hashicorp Vault, and modern deployment practices with Kubernetes and Istio.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Building and deploying microservices can be easy in Spring! Libraries like Spring Boot, Spring Cloud, and Spring Cloud Gateway reduce the boilerplate code in REST-based services. They provide an effective toolbox to get your microservices up and running on both public and private clouds.
About the book
Spring Microservices in Action, Second Edition teaches you to build microservice-based applications using Java and Spring. You’ll start by creating basic services, then move to efficient logging and monitoring. Learn to refactor Java applications with Spring’s intuitive tooling, and master API management with Spring Cloud Gateway. You’ll even deploy Spring Cloud applications with AWS and Kubernetes.
What's inside
Microservice design principles and best practices
Configuration with Spring Cloud Config and Hashicorp Vault
Client-side resiliency with Resilience4j, and Spring Cloud Load Balancer
Metrics monitoring with Prometheus and Grafana
Distributed tracing with Spring Cloud Sleuth, Zipkin, and ELK Stack
About the reader
For experienced Java and Spring developers.
About the author
John Carnell is a senior cloud engineer with 20 years of Java experience. Illary Huaylupo Sánchez is a software engineer with over 13 years of experience.
Table of Contents
1 Welcome to the cloud, Spring
2 Exploring the microservices world with Spring Cloud
3 Building microservices with Spring Boot
4 Welcome to Docker
5 Controlling your configuration with the Spring Cloud Configuration Server
6 On service discovery
7 When bad things happen: Resiliency patterns with Spring Cloud and Resilience4j
8 Service routing with Spring Cloud Gateway
9 Securing your microservices
10 Event-driven architecture with Spring Cloud Stream
11 Distributed tracing with Spring Cloud Sleuth and Zipkin
12 Deploying your microservices
Product Details
ISBN-13: | 9781638356967 |
---|---|
Publisher: | Manning |
Publication date: | 06/29/2021 |
Sold by: | SIMON & SCHUSTER |
Format: | eBook |
Pages: | 448 |
File size: | 20 MB |
Note: | This product may take a few minutes to download. |
About the Author
Illary Huaylupo Sánchez is a software engineer with an MBA in IT management and over twelve years of experience in Java.
Table of Contents
Preface xvii
Acknowledgments xix
About this book xxi
About the authors xxv
About the cover illustration xxvii
1 Welcome to the cloud, Spring 1
1.1 The evolution towards a microservices architecture 2
N-tier architecture 2
What's a monolithic architecture? 3
What's a microservice? 4
Why change the way we build applications? 6
1.2 Microservices with Spring 7
1.3 What are we building? 8
1.4 What is this book about? 10
What you'll learn in this book 10
Why is this book relevant to you? 10
1.5 Cloud and microservice-based applications 11
Building a microservice with Spring Boot 11
What exactly is cloud computing? 16
Why the cloud and microservices? 18
1.6 Microservices are more than writing the code 19
1.7 Core microservice development pattern 21
1.8 Microservice routing patterns 22
1.9 Microservice client resiliency 23
1.10 Microservice security patterns 24
1.11 Microservice logging and tracing patterns 26
1.12 Application metrics pattern 27
1.13 Microservice build/deployment patterns 28
2 Exploring the microservices world with Spring Cloud 31
2.1 What is Spring Cloud? 32
Spring Cloud Config 33
Spring Cloud Service Discovery 33
Spring Cloud LoadBalancer and Resilience4j 33
Spring Cloud API Gateway 34
Spring Cloud Stream 34
Spring Cloud Sleuth 34
Spring Cloud Security 35
2.2 Spring Cloud by example 35
2.3 How to build a cloud-native microservice 37
Codebase 40
Dependencies 40
Config 41
Backing services 42
Build, release, run 42
Processes 43
Port binding 43
Concurrency 44
Disposability 44
Dev/prod parity 44
Logs 45
Admin processes 45
2.4 Making sure our examples are relevant 46
2.5 Building a microservice with Spring Boot and Java 47
Setting up the environment 47
Getting started with the skeleton project 47
Booting your Spring Boot application: Writing the bootstrap class 52
3 Building microservices with Spring Boot 55
3.1 The architect's story: Designing the microservice architecture 56
Decomposing the business problem 56
Establishing service granularity 59
Defining the service interfaces 61
3.2 When not to use microservices 62
Complexity when building distributed systems 62
Server or container sprawl 63
Application type 63
Data transactions and consistency 63
3.3 The developer's tale: Building a microservice with Spring Boot and Java 63
Building the doorway into the microservice: The Spring Boot controller 64
Adding internationalization into the licensing service 74
Implementing Spring HATEOAS to display related links 78
3.4 The DevOps story: Building for the rigors of runtime 81
Service assembly: Packaging and deploying your microservices 83
Service bootstrapping: Managing configuration of your microservices 84
Service registration and discovery: How clients communicate with your microservices 85
Communicating a microservice's health 86
3.5 Pulling the perspectives together 89
4 Welcome to Docker 91
4.1 Containers or virtual machines? 92
4.2 What is Docker? 94
4.3 Docket-files 96
4.4 Docker Compose 97
4.5 Integrating Docker with our microservices 99
Building the Docker Image 99
Creating Docker images with Spring Boot 104
Launching the services with Docker Compose 107
5 Controlling your configuration with the Spring Cloud Configuration Server 110
5.1 On managing configuration (and complexity) 111
Your configuration management architecture 112
Implementation choices 114
5.2 Building our Spring Cloud Configuration Server 115
Setting up the Spring Cloud Config bootstrap class 120
Using the Spring Cloud Config Server with a filesystem 121
Setting up the configuration files for a service 122
5.3 Integrating Spring Cloud Config with a Spring Boot client 127
Setting up the licensing service Spring Cloud Config Service dependencies 128
Configuring the licensing service to use Spring Cloud Config 129
Wiring in a data source -using Spring Cloud Config Server 133
Directly reading properties using @ConfigurationProperties 137
Refreshing your properties using Spring Cloud Config Server 138
Using Spring Cloud Configuration Server with Git 139
Integrating Vault with the Spring Cloud Config service 141
Vault UI 141
5.4 Protecting sensitive configuration information 144
Setting up a symmetric encryption key 144
Encrypting and decrypting a property 146
5.5 Closing thoughts 147
6 On service discovery 148
6.1 Where's my service? 149
6.2 Service discovery in the cloud 152
The architecture of service discovery 153
Service discovery in action using Spring and Netflix Eureka 156
6.3 Building our Spring Eureka service 158
6.4 Registering services with Spring Eureka 162
Eureka's REST API 166
Eureka dashboard 167
6.5 Using service discovery to look up a service 169
Looking up service instances with Spring Discovery Client 171
Invoking services with a Load Balancer-aware Spring REST template 173
Invoking services with Netflix Feign client 174
7 When bad things happen: Resiliency patterns with Spring & Cloud and Resilience4j 177
7.1 What are client-side resiliency patterns? 178
Client-side load balancing 179
Circuit breaker 180
Fallback processing 180
Bulkheads 180
7.2 Why client resiliency matters 181
7.3 Implementing Resilience4j 185
7.4 Setting up the licensing service to use Spring Cloud and Resilience4j 186
7.5 Implementing a circuit breaker 187
Adding the circuit breaker to the organization service 191
Customizing the circuit breaker 192
7.6 Fallback processing 193
7.7 Implementing the bulkhead pattern 195
7.8 Implementing the retry pattern 198
7.9 Implementing the rate limiter pattern 200
7.10 ThreadLocal and Resilience4j 202
8 Service routing with Spring Cloud Gateway 208
8.1 What is a service gateway? 209
8.2 Introducing Spring Cloud Gateway 211
Setting up the Spring Boot gateway project 212
Configuring the Spring Cloud Gateway to communicate with Eureka 215
8.3 Configuring routes in Spring Cloud Gateway 216
Automated mapping of routes via service discovery 216
Manually mapping routes using service discovery 218
Dynamically reloading route configuration 221
8.4 The real power of Spring Cloud Gateway: Predicate and Filter Factories 222
Built-in Predicate Factories 223
Built-in Filter Factories 224
Custom filters 225
8.5 Building the pre-filter 228
8.6 Using the correlation ID in the services 231
UserContextFilter: Intercepting the incoming HTTP request 233
UserContext: Making the HTTP headers easily accessible to the service 234
Custom Rest Template and UserContextInterceptor: Ensuring that the correlation ID gets propagated 235
8.7 Building a post-filter receiving correlation ID 237
9 Securing your microservices 240
9.1 What is OAuth2? 241
9.2 Introduction to Keycloak 242
9.3 Starting small: Using Spring and Keycloak to protect a single endpoint 244
Adding Keycloak to Docker 245
Setting up Keycloak 246
Registering a client application 248
Configuring O-stoch users 253
Authenticating our O-stock users 255
9.4 Protecting the organization service using Keycloak 258
Adding the Spring Security and Keycloak JARs to the individual services 259
Configuring the service to point to our Keycloak server 260
Defining who and what can access the service 260
Propagating the access token 265
Parsing a custom field in a JWT 270
9.5 Some closing thoughts on microservice security 272
Use HTTPS secure sockets layer (SSL) for all service communication 273
Use a service gateway to access your microservices 273
Zone your services into a public API and private API 273
Limit the attack surface of your microservices by locking down unneeded network ports 274
10 Eventrdriven architecture with Spring Cloud Stream 275
10.1 The case for messaging, EDA, and microservices 276
Using a synchronous request-response approach to communicate state change 277
Using messaging to communicate state changes between services 279
Downsides of a messaging architecture 281
10.2 Introducing Spring Cloud Stream 282
10.3 Writing a simple message producer and consumer 284
Configuring Apache Kafka and Redis in Docker 285
Writing the message producer in the organization service 286
Writing the message consumer in the licensing service 293
Seeing the message service in action 296
10.4 A Spring Cloud Stream use case: Distributed caching 297
Using Redis to cache lookups 298
Defining custom channels 304
Distributed tracing with Spring Cloud Sleuth and Zipkin 307
11.1 Spring Cloud Sleuth and the correlation ID 309
Adding Spring Cloud Sleuth to licensing and organization 309
Anatomy of a Spring Cloud Sleuth trace 310
11.2 Log aggregation and Spring Cloud Sleuth 311
A Spring Cloud Sleuth/ELK Stack implementation in action 313
Configuring Logback in our services 314
Defining and running ELK Stack applications in Docker 318
Configuring Kibana 320
Searching for Spring Cloud Sleuth trace IDs in Kibana 323
Adding the correlation ID to the HTTP response with Spring Cloud Gateway 325
11.3 Distributed tracing with Zipkin 327
Setting up the Spring Cloud Sleuth and Zipkin dependencies 328
Configuring the services to point to Zipkin 328
Configuring a Zipkin server 328
Setting tracing levels 329
Using Zipkin to trace transactions 330
Visualizing a-more complex transaction 333
Capturing messaging traces 334
Adding custom spans 336
12 Deploying your microservices 340
12.1 The architecture of a build/deployment pipeline 342
12.2 Setting up O-stock's core infrastructure in the cloud 346
Creating the PostgreSQL database using Amazon RDS 348
Creating the Redis cluster in Amazon 351
12.3 Beyond the infrastructure: Deploying O-stock and ELK 353
Creating an EC2 with ELK 353
Deploying the ELK Stack in the EC2 instance 356
Creating an EKS cluster 357
12.4 Your build/deployment pipeline in action 364
12.5 Creating our build/deploy pipeline 366
Setting up GitHub 366
Enabling our services to build in Jenkins 367
Understanding and generating the pipeline script 372
Creating the Kubernetes pipeline scripts 374
12.6 Closing thoughts on the build/deployment pipeline 375
Appendix A Microservices architecture best practices 377
Appendix B OAuth2 grant types 383
Appendix C Monitoring your microservices 392
Index 403