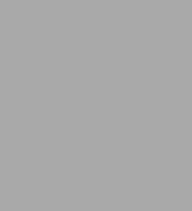
Reactive Programming with RxJava: Creating Asynchronous, Event-Based Applications
370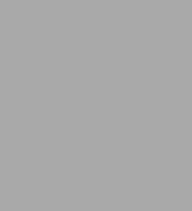
Reactive Programming with RxJava: Creating Asynchronous, Event-Based Applications
370Paperback
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
Related collections and offers
Overview
Authors Tomasz Nurkiewicz and Ben Christensen include concrete examples that use the RxJava library to solve real-world performance issues on Android devices as well as the server. You'll learn how RxJava leverages parallelism and concurrency to help you solve today's problems. This book also provides a preview of the upcoming 2.0 release.
- Write programs that react to multiple asynchronous sources of input without descending into "callback hell"
- Get to that aha! moment when you understand how to solve problems in the reactive way
- Cope with Observables that produce data too quickly to be consumed
- Explore strategies to debug and to test programs written in the reactive style
- Efficiently exploit parallelism and concurrency in your programs
- Learn about the transition to RxJava version 2
Product Details
ISBN-13: | 9781491931653 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 10/30/2016 |
Pages: | 370 |
Product dimensions: | 7.00(w) x 9.10(h) x 1.00(d) |
About the Author
Ben Christensen is a software engineer focused on resilience, scale and distributed systems. Open source projects created while addressing these requirements include Hystrix
(https://github.com/Netflix/Hystrix) and RxJava
(https://github.com/ReactiveX/RxJava).
Table of Contents
Foreword xiii
Introduction xvii
1 Reactive Programming with RxJava 1
Reactive Programming and Rxjava 1
When You Need Reactive Programming 3
How RxJava Works 4
Push versus Pull 4
Async versus Sync 5
Concurrency and Parallelism 8
Lazy versus Eager 12
Duality 13
Cardinality 15
Mechanical Sympathy: Blocking versus Nonblocking I/O 20
Reactive Abstraction 25
2 Reactive Extensions 27
Anatomy of rx.Observable 27
Subscribing to Notifications from Observable 30
Capturing All Notifications by Using Observer Controlling Listeners by Using Subscription and Subscriber Creating Observables 34 Mastering Gbservable.create() 35 Infinite Streams 38 Timing: timer() and interval() 43 Hot and Cold Observables 43 Use Case: From Callback API to Observable Stream 45 Manually Managing Subscribers 49 rx. subjects. Subject 51 ConnectableObservable 53 Single Subscription with publish().refCount() 54 ConnectableObservable Lifecycle 56 Summary 59 3 Operators and Transformations 61 Core Operators: Mapping and Filtering 61 1 -to-1 Transformations Using map() 64 Wrapping Up Using flatMap() 67 Postponing Events Using the delay() Operator 72 Order of Events After flatMap() 73 Preserving Order Using concatMap() 75 More Than One Observable 77 Treating Several Observables as One Using merge() 78 Pairwise Composing Using zip() and zipWith() 79 When Streams Are Not Synchronized with One Another: combineLatestf), withLatestFrom(), and amb() 83 Advanced Operators: collect(), reduce(), scan(), distinct(), and groupBy() 88 Scanning Through the Sequence with Scan and Reduce 88 Reduction with Mutable Accumulator: collect() 91 Asserting Observable Has Exactly One Item Using singleQ 92 Dropping Duplicates Using distinct() and distincfUntilChanged() 92 Slicing and Dicing Using skip(), takeWhileQ, and Others 94 Ways of Combining Streams: concat(), merge(), and switchOnNext() 97 Criteria-Based Splitting of Stream Using groupBy() 104 Where to Go from Here? 107 Writing Customer Operators 107 Reusing Operators Using compose() 108 Implementing Advanced Operators Using lift() 110 Summary 115 4 Applying Reactive Programming to Existing Applications 117 From Collections to Observables 118 BlockingObservable: Exiting the Reactive World 118 Embracing Laziness 121 Composing Observables 123 Lazy paging and concatenation 124 Imperative Concurrency 125 FlatMap() as Asynchronous Chaining Operator 131 Replacing Callbacks with Streams 135 Polling Periodically for Changes 138 Multithreading in RxJava 140 What Is a Scheduler? 141 Declarative Subscription with subscribeOn() 150 SubscribeOn() Concurrency and Behavior 154 Batching Requests Using groupByQ 158 Declarative Concurrency wim observeOn() 159 Other Uses for Schedulers 163 Summary 164 5 Reactive from Top to Bottom 165 Beating the C10k Problem 165 Traditional Thread-Based HTTP Servers 167 Nonblocking HTTP Server with Netty and RxNetty 169 Benchmarking Blocking versus Reactive Server 177 Reactive HTTP Servers Tour 183 HTTP Client Code 184 Nonblocking HTTP Client with RxNetty 184 Relational Database Access 187 NOTIFY AND LISTEN on PostgreSQL Case Study 189 CompletableFuture and Streams 193 A Short Introduction to CompletableFuture 193 Interoperability with CompletableFuture 198 Observable versus Single 202 Creating and Consuming Single 203 Combining Responses Using zip, merge, and concat 205 Interoperability with Observable and CompletableFuture 207 When to Use Single? 208 Summary 209 6 Flow Control and Backpressure 211 Flow Control 211 Taking Periodic Samples and Throttling 212 Buffering Events to a List 214 Moving window 220 Skipping Stale Events by Using debounce() 221 Backpressure 226 Backpressure in Rxjava 227 Built-in Backpressure 231 Producers and Missing Backpressure 233 Honoring the Requested Amount of Data 237 Summary 242 7 Testing and Troubleshooting 243 Error Handling 243 Where Are My Exceptions? 244 Declarative try-catch Replacement 247 Timing Out When Events Do Not Occur 251 Retrying After Failures 254 Testing and Debugging 258 Virtual Time 258 Schedulers in Unit Testing 260 Unit Testing 262 Monitoring and Debugging 270 DoOn…() Callbacks 270 Measuring and Monitoring 272 Summary 275 8 Case Studies 277 Android Development with Rxjava 277 Avoiding Memory Leaks in Activities 278 Retrofit with Native Rxjava Support 280 Schedulers in Android 285 UI Events as Streams 288 Managing Failures with Hystrix 291 The First Steps with Hystrix 292 Nonblocking Commands with HystrixObservable Command 294 Bulkhead Pattern and Fail-Fast 295 Batching and Collapsing Commands 297 Monitoring and Dashboards 303 Querying NoSQL Databases 306 Couchbase Client API 306 MongoDB Client API 307 Camel Integration 309 Consuming Files with Camel 309 Receiving Messages from Kafka 310 Java 8 Streams and CompletableFuture 310 Usefulness of Parallel Streams 312 Choosing the Appropriate Concurrency Abstraction 314 When to Choose Observable? 315 Memory Consumption and Leaks 315 Operators Consuming Uncontrolled Amounts of Memory 316 Summary 321 9 Future Directions 323 Reactive Streams 323 Observable and Flowable 323 Performance 324 Migration 325 A More HTTP Server Examples 327 B A Decision Tree of Observable Operators 333 Index 339