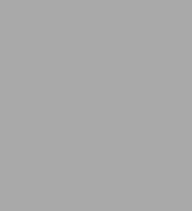
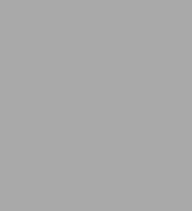
Paperback(2nd ed.)
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
Related collections and offers
Overview
Through the course of this book, author Stoyan Stefanov helps web developers and programmers build a complete single-page application. You'll quickly learn why some developers consider React the key to the web app development puzzle.
- Set up React and write your first "Hello, World" web app
- Create and use custom React components alongside generic DOM components
- Build a data table component that lets you edit, sort, search, and export its contents
- Master the JSX syntax
- Use built-in Hooks and create your own custom ones
- Manage the app's data flow with reducers and contexts
- Use Create React App to take care of the build process and focus on React itself
- Build a complete custom app that lets you store data on the client
Product Details
ISBN-13: | 9781492051466 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 12/21/2021 |
Edition description: | 2nd ed. |
Pages: | 230 |
Sales rank: | 700,137 |
Product dimensions: | 6.90(w) x 9.10(h) x 0.60(d) |
About the Author
Table of Contents
Preface xi
1 Hello World 1
Setup 1
Hello React World 2
What Just Happened? 4
React.DOM.* 5
Special DOM Attributes 8
React DevTools Browser Extension 9
Next: Custom Components 10
2 The Life of a Component 11
Bare Minimum 11
Properties 13
PropTypes 14
Default Property Values 17
State 18
A Stateful Textarea Component 18
A Note on DOM Events 22
Event Handling in the Olden Days 22
Event Handling in React 24
Props Versus State 24
Props in Initial State: An Anti-Pattern 24
Accessing the Component from the Outside 25
Changing Properties Mid-Flight 27
Lifecycle Methods 28
Lifecycle Example: Log It All 29
Lifecycle Example: Use a Mixin 32
Lifecycle Example: Using a Child Component 33
Performance Win: Prevent Component Updates 36
PureRenderMixin 39
3 Excel: A Fancy Table Component 41
Data First 41
Table Headers Loop 42
Debugging the Console Warning 44
Adding <td> Content 46
How Can You Improve the Component? 48
Sorting 48
How Can You Improve the Component? 50
Sorting UI Cues 50
Editing Data 51
Editable Cell 52
Input Field Cell 54
Saving 54
Conclusion and Virtual DOM Diffs 55
Search 56
State and UI 58
Filtering Content 60
How Can You Improve the Search? 62
Instant Replay 63
How Can You Improve the Replay? 64
An Alternative Implementation? 64
Download the Table Data 65
4 JSX 67
Hello JSX 67
Transpiring JSX 68
Babel 69
Client Side 69
About the JSX transformation 71
JavaScript in JSX 73
Whitespace in JSX 75
Comments in JSX 76
HTML Entities 77
Anti-XSS 78
Spread Attributes 79
Parent-to-Child Spread Attributes 79
Returning Multiple Nodes in JSX 81
JSX Versus HTML Differences 83
No class, What for? 83
Style Is an Object 83
Closing Tags 83
CamelCase Attributes 84
JSX and Forms 84
OnChange Handler 84
Value Versus defaultValue 85
<Textarea> Value 85
<Select> Value 87
Excel Component in JSX 88
5 Setting Up for App Development 89
A Boilerplate App 89
Files and Folders 90
Index.html 91
CSS 92
JavaScript 92
JavaScript: Modernized 93
Installing Prerequisites 96
Node.js 97
Browserify 97
Babel 97
React, etc. 98
Let's Build 98
Transpile JavaScript 98
Package JavaScript 98
Package CSS 99
Results! 99
Windows Version 99
Building During Development 99
Deployment 100
Moving On 101
6 Building an App 103
Whinepad v.0.0.1 103
Setup 103
Start Coding 104
The Components 106
Setup 106
Discover 106
<Button> Component 108
Button.css 109
Button.Js 109
Forms 113
<Suggest> 113
<Rating> Component 116
A <FormInput> "Factory" 119
<Form> 121
<Actions> 124
Dialogs 125
App Config 128
<Excel>: New and Improved 129
<Whinepad> 138
Wrapping It All Up 142
7 Lint, Flow, Test, Repeat 143
Package.json 143
Configure Babel 144
Scripts 144
ESLint 145
Setup 145
Running 145
All the Rules 147
Flow 147
Setup 147
Running 148
Signing Up for Typechecking 148
Fixing <Button> 149
App.js 150
More on Typechecking props and state 152
Export/Import Types 153
Typecasting 154
Invariants 155
Testing 156
Setup 157
First Test 158
First React Test 159
Testing the <Button> Component 160
Testing <Actions> 163
More Simulated Interactions 165
Testing Complete Interactions 167
Coverage 169
8 Flux 173
The Big Idea 174
Another Look at Whinepad 174
The Store 175
Store Events 177
Using the Store in <Whinepad> 179
Using the Store in <Excel> 181
Using the Store in <Form> 182
Drawing the Line 183
Actions 183
CRUD Actions 183
Searching and Sorting 184
Using the Actions in <Whinepad> 186
Using the Actions in <Excel> 188
Flux Recap 190
Immutable 191
Immutable Store Data 192
Immutable Data Manipulation 193
Index 197