Programming with POSIX Threads / Edition 1 available in Paperback, eBook
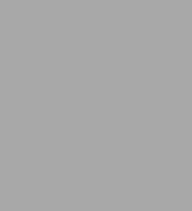
Programming with POSIX Threads / Edition 1
- ISBN-10:
- 0201633922
- ISBN-13:
- 9780201633924
- Pub. Date:
- 05/16/1997
- Publisher:
- Pearson Education
- ISBN-10:
- 0201633922
- ISBN-13:
- 9780201633924
- Pub. Date:
- 05/16/1997
- Publisher:
- Pearson Education
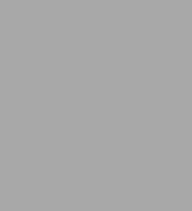
Programming with POSIX Threads / Edition 1
Buy New
$64.99Buy Used
$18.93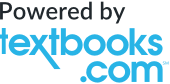
-
SHIP THIS ITEM— This item is available online through Marketplace sellers.
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
This item is available online through Marketplace sellers.
-
SHIP THIS ITEM
Temporarily Out of Stock Online
Please check back later for updated availability.
This item is available online through Marketplace sellers.
Overview
The primary advantage of threaded programming is that it enables your applications to accomplish more than one task at the same time by using the number-crunching power of multiprocessor parallelism and by automatically exploiting I/O concurrency in your code, even on a single processor machine. The result: applications that are faster, more responsive to users, and often easier to maintain. Threaded programming is particularly well suited to network programming where it helps alleviate the bottleneck of slow network I/O.
This book offers an in-depth description of the IEEE operating system interface standard, POSIXAE (Portable Operating System Interface) threads, commonly called Pthreads. Written for experienced C programmers, but assuming no previous knowledge of threads, the book explains basic concepts such as asynchronous programming, the lifecycle of a thread, and synchronization. You then move to more advanced topics such as attributes objects, thread-specific data, and realtime scheduling. An entire chapter is devoted to "real code," with a look at barriers, read/write locks, the work queue manager, and how to utilize existing libraries. In addition, the book tackles one of the thorniest problems faced by thread programmers-debugging-with valuable suggestions on how to avoid code errors and performance problems from the outset.
Numerous annotated examples are used to illustrate real-world concepts. A Pthreads mini-reference and a look at future standardization are also included.
Product Details
ISBN-13: | 9780201633924 |
---|---|
Publisher: | Pearson Education |
Publication date: | 05/16/1997 |
Series: | Addison-Wesley Professional Computing Series |
Edition description: | New Edition |
Pages: | 400 |
Product dimensions: | 7.30(w) x 9.10(h) x 0.90(d) |
About the Author
0201633922AB04062001
Read an Excerpt
The White Rabbit put on his spectacles,
"Where shall I begin, please your Majesty?" he asked.
"Begin at the beginning," the King said, very gravely,
"and go on till you come to the end: then stop."
 ***-Lewis Carroll, Alice's Adventures in Wonderland
This book is about "threads" and how to use them. "Thread" is just a name for a basic software "thing" that can do work on a computer. A thread is smaller, faster, and more maneuverable than a traditional process. In fact, once threads have been added to an operating system, a "process" becomes just dataaddress space, files, and so forthplus one or more threads that do something with all that data.
With threads, you can build applications that utilize system resources more efficiently, that are more friendly to users, that run blazingly fast on multiprocessors, and that may even be easier to maintain. To accomplish all this, you need only add some relatively simple function calls to your code, adjust to a new way of thinking about programming, and leap over a few yawning chasms. Reading this book carefully will, I hope, help you to accomplish all that without losing your sense of humor.
The threads model used in this book is commonly called "Pthreads," or "POSIX threads." Or, more formally (since you haven't yet been properly introduced), the POSIX 1003.1cn1995 standard. I'll give you a few other names later-but for now, "Pthreads" is all you need to worry about.
As I write this, Sun's Solaris, Digital's Digital UNIX, and SGI's IRIX already support Pthreads. The other major commercial UNIX operating systems will soon have Pthreads as well, maybe even by the time you read this, including IBM's AIX and Hewlett-Packard's HP-UX. Pthreads implementations are also available for Linux and other UNIX operating systems.
In the personal computer market, Microsoft's Win32 API (the primary programming interface to both Windows NT and Windows 95) supports threaded programming, as does IBM's OS/2. These threaded programming models are quite different from Pthreads, but the important first step toward using them productively is understanding concurrency, synchronization, and scheduling. The rest is (more or less) a matter of syntax and style, and an experienced thread programmer can adapt to any of these models.
The threaded model can be (and has been) applied with great success to a wide range of programming problems. Here are just a few:
- Large scale, computationally intensive programs
- High-performance application programs and library code that can take advantage of multiprocessor systems
- Library code that can be used by threaded application programs
- Realtime application programs and library code
- Application programs and library code that perform I/O to slow external devices (such as networks and human beings).
This book assumes that you are an experienced programmer, familiar with developing code for an operating system in "the UNIX family" using the ANSI C language. I have tried not to assume that you have any experience with threads or other forms of asynchronous programming. The Introduction chapter provides a general overview of the terms and concepts you'll need for the rest of the book. If you don't want to read the Introduction first, that's fine, but if you ever feel like you're "missing something" you might try skipping back to get introduced.
Along the way you'll find examples and simple analogies for everything. In the end I hope that you'll be able to continue comfortably threading along on your own. Have fun, and "happy threading."
About the author
I have been involved in the Pthreads standard since it began, although I stayed at home for the first few meetings. I was finally forced to spend a grueling week in the avalanche-proof concrete bunker at the base of Snowbird ski resort in Utah, watching hard-working standards representatives from around the world wax their skis. This was very distracting, because I had expected a standards meeting to be a formal and stuffy environment. As a result of this misunderstanding, I was forced to rent ski equipment instead of using my own.
After the Pthreads standard went into balloting, I worked on additional thread synchronization interfaces and multiprocessor issues with several POSIX working groups. I also helped to define the Aspen threads extensions, which were fast-tracked into X/Open XSH5.
I have worked at Digital Equipment Corporation for (mumble, mumble) years, in various locations throughout Massachusetts and New Hampshire. I was one of the creators of Digital's own threading architecture, and I designed (and implemented much of) the Pthreads interfaces on Digital UNIX 4.0. I have been helping people develop and debug threaded code for more than eight years.
My unofficial motto is "Better Living Through Concurrency." Threads are not sliced bread, but then, we're programmers, not bakers, so we do what we can.
Acknowledgments
This is the part where I write the stuff that I'd like to see printed, and that my friends and coworkers want to see. You probably don't care, and I promise not to be annoyed if you skip over it-nbut if you're curious, by all means read on.
No project such as this book can truly be accomplished by a single person, despite the fact that only one name appears on the cover. I could have written a book about threads without any help-I know a great deal about threads, and I am at least reasonably competent at written communication. However, the result would not have been this book, and this book is better than that hypothetical work could possibly have been.
Thanks first and foremost to my manager Jean Fullerton, who gave me the time and encouragement to write this book on the job-and thanks to the rest of the DECthreads team who kept things going while I wrote, including Brian Keane, Webb Scales, Jacqueline Berg, Richard Love, Peter Portante, Brian Silver, Mark Simons, and Steve Johnson.
Thanks to Garret Swart who, while he was with Digital at the Systems Research Center, got us involved with POSIX. Thanks to Nawaf Bitar who worked with Garret to create, literally overnight, the first draft of what became Pthreads, and who became POSIX thread evangelist through the difficult period of getting everyone to understand just what the heck this threading thing was all about anyway. Without Garret, and especially Nawaf, Pthreads might not exist, and certainly wouldn't be as good as it is. (The lack of perfection is not their responsibility-that's the way life is.)
Thanks to everyone who contributed to the design of cma, Pthreads, UNIX98, and to the users of DCE threads and DECthreads, for all the help, thought-provoking discourse, and assorted skin-thickening exercises, including Andrew Birrell, Paul Borman, Bob Conti, Bill Cox, Jeff Denham, Peter Gilbert, Rick Greer, Mike Grier, Kevin Harris, Ken Hobday, Mike Jones, Steve Kleiman, Bob Knighten, Leslie Lamport, Doug Locke, Paula Long, Finnbarr P. Murphy, Bill Noyce, Simon Patience, Harold Seigel, Al Simons, Jim Woodward, and John Zolnowsky.
Many thanks to all those who patiently reviewed the drafts of this book (and even to those who didn't seem so patient at times). Brian Kernighan, Rich Stevens, Dave Brownell, Bill Gallmeister, Ilan Ginzburg, Will Morse, Bryan O'Sullivan, Bob Robillard, Dave Ruddock, Bil Lewis, and many others suggested or motivated improvements in structure and detail-and provided additional skin-thickening exercises to keep me in shape. Devang Shah and Bart Smaalders answered some Solaris questions, and Bryan O'Sullivan suggested what became the "bailing programmers" analogy.
Thanks to John Wait and Lana Langlois at Addison Wesley Longman, who waited with great patience as a first-time writer struggled to balance writing a book with engineering and consulting commitments. Thanks to Pamela Yee and Erin Sweeney, who managed the book's production process, and to all the team (many of whose names I'll never know), who helped. Thanks to my wife, Anne Lederhos, and our daughters Amy and Alyssa, for all the things for which any writers may thank their families, including support, tolerance, and just being there. And thanks to Charles Dodgson (Lewis Carroll), who wrote extensively about threaded programming (and nearly everything else) in his classic works Alice's Adventures in Wonderland, Through the Looking-Glass, and The Hunting of the Snark.
Dave Butenhof Digital Equipment Corporation
110 Spit Brook Road, ZKO2-3/Q18
Nashua, NH 03062
butenhof@zko.dec.com December 1996
Table of Contents
List of Example Programs.Preface.
Intended Audience.
About the Author.
Acknowledgments.
1. Introduction.
The "Bailing Programmers".
Definitions and Terminology.
Asynchronous.
Concurrency.
Uniprocessor and Multiprocessor.
Parallelism.
Thread Safety and Reentrancy.
Concurrency Control Functions.
Asynchronous Programming is Intuitive...
. . . Because Unix is Asynchronous.
. . . Because the World is Asynchronous.
About the Examples in This Book.
Asynchronous Programming, by Example.
the Baseline, Synchronous Version.
A Version Using Multiple Processes.
A Version Using Multiple Threads.
Summary.
Benefits of Threading.
Parallelism.
Concurrency.
Programming Model.
Costs of Threading.
Computing Overhead.
Programming Discipline.
Harder to Debug.
To Thread or Not to Thread?
POSIX Thread Concepts.
Architectural Overview.
Types and Interfaces.
Checking for Errors.
2. Threads.
Creating and Using Threads.
The Life of a Thread.
Creation.
Startup.
Running and Blocking.
Termination.
Recycling.
3. Synchronization.
Invariants, Critical Sections, and Predicates.
Mutexes.
Creating and Destroying a Mutex.
Locking and Unlocking a Mutex.
Nonblocking Mutex Locks.
Using Mutexes for Atomicity.
Sizing a Mutex to Fit the Job.
Using More Than One Mutex.
Lock Hierarchy.
Lock Chaining.
Condition Variables.
Creating and Destroying a Condition Variable.
Waiting on a Condition Variable.
Waking Condition Variable Waiters.
One Final Alarm Program.
Memory Visibility Between Threads.
4. A Few Ways to Use Threads.
Pipeline.
Work Crew.
Client/Server.
5. Advanced Threaded Programming.
One-Time Initialization.
Attributes Objects.
Mutex Attributes.
Condition Variable Attributes.
Thread Attributes.
Cancellation.
Deferred Cancelability.
Asynchronous Cancelability.
Cleaning Up.
Thread-Specific Data.
Creating Thread-Specific Data.
Using Thread-Specific Data.
Using Destructor Functions.
Realtime Scheduling.
POSIX Realtime Options.
Scheduling Policies and Priorities.
Contention Scope and Allocation Domain.
Problems With Realtime Scheduling.
Priority-Aware Mutexes.
Priority Ceiling Mutexes.
Priority Inheritance Mutexes.
Threads and Kernel Entities.
Many-to-One (User Level).
One-to-One (Kernel Level).
Many-to-Few (Two Level).
6. Posix Adjusts to Threads.
Fork.
Fork Handlers.
Exec.
Process Exit.
Stdio.
Flockfile and Funlockfile.
Getchar_Unlocked and Putchar_Unlocked.
Thread-Safe Functions.
User and Terminal Identification.
Directory Searching.
String Token.
Time Representation.
Random Number Generation.
Group and User Database.
Signals.
Signal Actions.
Signal Masks.
Pthread_Kill.
Sigwait and Sigwaitinfo.
Sigev_Thread.
Semaphores: Synchronizing With a Signal-Catching Function.
7. "Real Code".
Extended Synchronization.
Barriers.
Read-Write Locks.
Work Queue Manager.
But What About Existing Libraries?
Modifying Libraries to Be Thread-Safe.
Living With Legacy Libraries.
8. Hints to Avoid Debugging.
Avoiding Incorrect Code.
Avoid Relying on "Thread Inertia".
Never Bet Your Mortgage on a Thread Race.
Cooperate to Avoid Deadlocks.
Beware of Priority Inversion.
Never Share Condition Variables Between Predicates.
Sharing Stacks and Related Memory Corrupters.
Avoiding Performance Problems.
Beware of Concurrent Serialization.
Use the Right Number of Mutexes.
Too Many Mutexes Will Not Help.
Never Fight Over Cache Lines.
9. Posix Threads Mini-Reference.
POSIX 1003.1cn1995 Options.
POSIX 1003.1cn1995 Limits.
POSIX 1003.1cn1995 Interfaces.
Error Detection and Reporting.
Use of Void* Type.
Threads.
Mutexes.
Condition Variables.
Cancellation.
Thread-Specific Data.
Realtime Scheduling.
Fork Handlers.
Stdio.
Thread-Safe Functions.
Signals.
Semaphores.
10. Future Standardization.
X/Open Xsh5 [Unix98].
POSIX Options for XSH5.
Mutex Type.
Set Concurrency Level.
Stack Guard Size.
Parallel I/O.
Cancellation Points.
POSIX 1003.1j.
Barriers.
Read-Write Locks.
Spinlocks.
Condition Variable Wait Clock.
Thread Abort.
Posix 1003.14.
Bibliography.
Thread Resources on the Internet.
Index. 0201633922T04062001