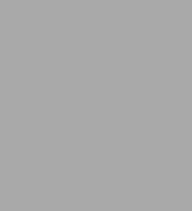
Microservices in .NET Core: with examples in Nancy
344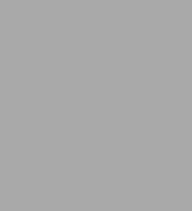
Microservices in .NET Core: with examples in Nancy
344Paperback(1st Edition)
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
Related collections and offers
Overview
Microservices in .NET Core provides a complete guide to building microservice applications. After a crystal-clear introduction to the microservices architectural style, the book will teach you practical development skills in that style, using OWIN and Nancy.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Microservice applications are built by connecting single-capability, autonomous components that communicate via APIs. These systems can be challenging to develop because they demand clearly defined interfaces and reliable infrastructure. Fortunately for .NET developers, OWIN (the Open Web Interface for .NET), and the Nancy web framework help minimize plumbing code and simplify the task of building microservice-based applications.
About the Book
Microservices in .NET Core provides a complete guide to building microservice applications. After a crystal-clear introduction to the microservices architectural style, the book will teach you practical development skills in that style, using OWIN and Nancy. You'll design and build individual services in C# and learn how to compose them into a simple but functional application back end. Along the way, you'll address production and operations concerns like monitoring, logging, and security.
What's Inside
- Design robust and ops-friendly services
- Build HTTP APIs with Nancy
- Expose events via feeds with Nancy
- Use OWIN middleware for plumbing
About the Reader
This book is written for C# developers. No previous experience with microservices required.
About the Author
Christian Horsdal Gammelgaard is a Nancy committer and a Microsoft MVP.
Table of Contents
- Microservices at a glance
- A basic shopping cart microservice
- Identifying and scoping microservices
- Microservice collaboration
- Data ownership and data storage
- Designing for robustness
- Writing tests for microservices
- Introducing OWIN: writing and testing OWINmiddleware
- Cross-cutting concerns: monitoring and logging
- Securing microservice-to-microservicecommunication
- Building a reusable microservice platform
- Creating applications over microservices
Product Details
ISBN-13: | 9781617293375 |
---|---|
Publisher: | Manning |
Publication date: | 02/03/2017 |
Edition description: | 1st Edition |
Pages: | 344 |
Product dimensions: | 7.30(w) x 9.20(h) x 0.90(d) |
About the Author
Table of Contents
Preface xiii
Acknowledgments xiv
About this book xv
About the cover illustration xix
Part 1 Getting Started with Microservices 1
1 Microservices at a glance 3
1.1 What is a microservice? 3
What is a microservices architecture? 5
Microservice characteristics 5
1.2 Why microservices? 10
Enabling continuous delivery 11
High level of maintainability 12
Robust and scalable 13
1.3 Costs and downsides of microservices 13
1.4 Greenfield vs. brownfield 14
1.5 Code reuse 15
1.6 Serving a user request: an example of how microservices work in concert 16
Main handling of the user request 17
Side effects of the user request 18
The complete picture 19
1.7 A .NET microservices technology stack 20
Nancy 20
OWIN 21
Setting up a development environment 22
1.8 A simple microservices example 23
Creating an empty ASP.NET Core application 24
Adding Nancy to the project 24
Adding a Nancy module with an implementation of the endpoint 25
Adding OWIN middleware 27
1.9 Summary 28
2 A basic shopping cart microservice 30
2.1 Overview of the Shopping Carr microservice 31
Components of the Shopping Cart microservice 33
2.2 Implementing the Shopping Cart microservice 34
Creating an empty project 34
The Shopping Cart microservice's API for other services 35
Fetching product information 42
Parsing the product response 44
Adding a failure-handling policy 46
Implementing a basic event feed 48
2.3 Running the code 52
2.4 Summary 52
Part 2 Building Microservices 55
3 Identifying and scoping microservices 57
3.1 The primary driver for scoping microservices: business capabilities 58
What is a business capability? 58
Identifying business capabilities 59
Example: point-of-sale system 60
3.2 The secondary driver for scoping microservices: supporting technical capabilities 65
What is a technical capability? 65
Examples of supporting technical capabilities 65
Identifying technical capabilities 69
3.3 What to do when the correct scope isn't clear 69
Starting a bit bigger 70
Carving out new microservices from existing microservices 73
Planning to cane out new microservices later 75
3.4 Well-scoped microservices adhere to the microservice characteristics 75
Primarily scoping to business capabilities leads to good microservices 76
Secondarily scoping to supporting technical capabilities leads to good microservices 76
3.5 Summary 77
4 Microservice collaboration 79
4.1 Types of collaboration: commands, queries, and events 80
Commands and queries: synchronous collaboration 82
Events: asynchronous collaboration 85
Data formats 87
4.2 Implementing collaboration 88
Setting up a project for Loyalty Program 89
Implementing commands and queries 91
Implementing commands with HTTP POST or PUT 91
Implementing queries with HTTP GET 95
Data formats 96
Implementing an event-based, collaboration 98
4.3 Summary 107
5 Data ownership and data storage 109
5.1 Each microservice has a data store 110
5.2 Partitioning data between microservices 110
Rule 1 Ownership of data follows business capabilities 110
Rule 2 Replicate for speed and robustness 113
Where does a microservice store its data? 116
5.3 Implementing data storage in a microservice 118
Storing data owned by a microservice 119
Storing events raised by a microservice 122
Setting cache headers in Nancy responses 129
Reading and using cache headers 130
5.4 Summary 132
6 Designing for robustness 134
6.1 Expect failures 135
Keeping good logs 136
Using correlation tokens 138
Rolling forward vs. rolling back 138
Don't propagate failures 139
6.2 The client side's responsibility for robustness 140
Robustness pattern: retry 142
Robustness pattern: circuit breaker 144
6.3 Implementing robustness patterns 146
Implementing a fast-paced retry strategy with Polly 148
Implementing a circuit breaker with Polly 149
Implementing a slow-paced retry strategy 150
Logging all unhandled exceptions 153
6.4 Summary 154
7 Writing tests for microservices 155
7.1 What and how to test 156
The test pyramid: what to test in a microservices system 156
System-level tests: testing a complete microservice system end-to-end 157
Service-level tests: testing a microservice from outside its process 158
Unit-level tests: testing endpoints from within the process 161
7.2 Testing libraries: Nancy.Testing and xUnit 162
Meet Nancy. Testing 162
Meet xUnit 163
xUnit and Nancy.Testing working together 163
7.3 Writing unit tests using Nancy.Testing 164
Setting up a unit-test project 165
Using the Browser object to unit-test endpoints 167
Using a configurable bootstrapper to inject mocks into endpoints 170
7.4 Writing service-level tests 173
Creating a service-level test project 175
Creating mocked endpoints 175
Starling all the processes of the microservice under test 177
Executing the test scenario against the microservice under test 179
7.5 Summary 180
Part 3 Handling Cross-Cutting Concerns: Building a Reusable Microservice Platform 183
8 Introducing OWIN: writing and testing OWIN middleware 185
8.1 Handling cross-cutting concerns 186
8.2 The OWIN pipeline 188
What belongs in OWIN, and what belongs in Nancy? 191
8.3 Writing middleware 192
Middleware as lambdas 193
Middleware classes 194
8.4 Testing middleware and pipelines 195
8.5 Summary 198
9 Cross-cutting concerns: monitoring and logging 199
9.1 Monitoring needs in microservices 200
9.2 Logging needs in microservices 203
Structured logging with Serilog 205
9.3 Implementing the monitoring middleware 206
Implementing the shallow monitoring endpoint 207
Implementing the deep monitoring endpoint 208
Adding the monitoring middleware to the OWIN pipeline 210
9.4 Implementing the logging middleware 212
Adding correlation tokens to all log messages 214
Adding a correlation token to all outgoing HTTP requests 215
Logging requests and request performance 219
Configuring an OWIN pipeline with a correlation token and logging middleware 220
9.5 Summary 222
10 Securing microservice-to-microservice communication 223
10.1 Microservice security concerns 224
Authenticating users at the edge 225
Authorizing users in microservices 226
How much should microservices trust each other? 227
10.2 Implementing secure microservice-to-microservice communication 229
Meet Identity Server 231
Implementing authentication with IdentityServer middleware 237
Implementing microservice-to-microservice authorization with Identity Server and middleware 239
Implementing user authorization in Nancy modules 242
10.3 Summary 246
11 Building a reusable microservice platform 248
11.1 Creating a new microservice should be quick and easy 249
11.2 Creating a reusable microservice platform 249
11.3 Packaging and sharing middleware with NuGet 251
Creating a package with logging and monitoring middleware 252
Creating a package with authorization, middleware 259
Creating a package with rest client factory 262
Automatically registering an HTTP client factory in Nancy's container 265
Using the microservice platform 267
11.4 Summary 270
Part 4 Building Applications 271
12 Creating applications over microservices 273
12.1 End user applications for microservice systems: one or many applications? 274
General-purpose applications 274
Specialized applications 275
12.2 Patterns for building applications over microservices 276
Composite applications: integrating at the frontend 276
API gateway 279
Backend for frontend (BFF) pattern 281
When to use each pattern 282
Client-side or sewer-side rendering? 283
12.3 Example: a shopping cart and product list 284
Creating an API gateway 287
Creating the product list GUI 289
Creating the shopping cart GUI 294
Letting users add products to the shopping cart 297
Letting users remove products from the shopping cart 299
12.4 Summary 300
Appendix A Development environment setup 303
Appendix B Deploying to production 308
Further reading 312
Index 315