Go Web Programming
Summary
Go Web Programming teaches you how to build scalable, high-performance web applications in Go using modern design principles.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
The Go language handles the demands of scalable, high-performance web applications by providing clean and fast compiled code, garbage collection, a simple concurrency model, and a fantastic standard library. It's perfect for writing microservices or building scalable, maintainable systems.
About the Book
Go Web Programming teaches you how to build web applications in Go using modern design principles. You'll learn how to implement the dependency injection design pattern for writing test doubles, use concurrency in web applications, and create and consume JSON and XML in web services. Along the way, you'll discover how to minimize your dependence on external frameworks, and you'll pick up valuable productivity techniques for testing and deploying your applications.
What's Inside
About the Reader
This book assumes you're familiar with Go language basics and the general concepts of web development.
About the Author
Sau Sheong Chang is Managing Director of Digital Technology at Singapore Power and an active contributor to the Ruby and Go communities.
Table of Contents
"1124386642"
Go Web Programming teaches you how to build scalable, high-performance web applications in Go using modern design principles.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
The Go language handles the demands of scalable, high-performance web applications by providing clean and fast compiled code, garbage collection, a simple concurrency model, and a fantastic standard library. It's perfect for writing microservices or building scalable, maintainable systems.
About the Book
Go Web Programming teaches you how to build web applications in Go using modern design principles. You'll learn how to implement the dependency injection design pattern for writing test doubles, use concurrency in web applications, and create and consume JSON and XML in web services. Along the way, you'll discover how to minimize your dependence on external frameworks, and you'll pick up valuable productivity techniques for testing and deploying your applications.
What's Inside
- Basics
- Testing and benchmarking
- Using concurrency
- Deploying to standalone servers, PaaS, and Docker
- Dozens of tips, tricks, and techniques
About the Reader
This book assumes you're familiar with Go language basics and the general concepts of web development.
About the Author
Sau Sheong Chang is Managing Director of Digital Technology at Singapore Power and an active contributor to the Ruby and Go communities.
Table of Contents
- PART 1 GO AND WEB APPLICATIONS
- Go and web applications
- Go ChitChat PART 2 BASIC WEB APPLICATIONS
- Handling requests
- Processing requests
- Displaying content
- Storing data PART 3 BEING REAL
- Go web services
- Testing your application
- Leveraging Go concurrency
- Deploying Go
Go Web Programming
Summary
Go Web Programming teaches you how to build scalable, high-performance web applications in Go using modern design principles.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
The Go language handles the demands of scalable, high-performance web applications by providing clean and fast compiled code, garbage collection, a simple concurrency model, and a fantastic standard library. It's perfect for writing microservices or building scalable, maintainable systems.
About the Book
Go Web Programming teaches you how to build web applications in Go using modern design principles. You'll learn how to implement the dependency injection design pattern for writing test doubles, use concurrency in web applications, and create and consume JSON and XML in web services. Along the way, you'll discover how to minimize your dependence on external frameworks, and you'll pick up valuable productivity techniques for testing and deploying your applications.
What's Inside
About the Reader
This book assumes you're familiar with Go language basics and the general concepts of web development.
About the Author
Sau Sheong Chang is Managing Director of Digital Technology at Singapore Power and an active contributor to the Ruby and Go communities.
Table of Contents
Go Web Programming teaches you how to build scalable, high-performance web applications in Go using modern design principles.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
The Go language handles the demands of scalable, high-performance web applications by providing clean and fast compiled code, garbage collection, a simple concurrency model, and a fantastic standard library. It's perfect for writing microservices or building scalable, maintainable systems.
About the Book
Go Web Programming teaches you how to build web applications in Go using modern design principles. You'll learn how to implement the dependency injection design pattern for writing test doubles, use concurrency in web applications, and create and consume JSON and XML in web services. Along the way, you'll discover how to minimize your dependence on external frameworks, and you'll pick up valuable productivity techniques for testing and deploying your applications.
What's Inside
- Basics
- Testing and benchmarking
- Using concurrency
- Deploying to standalone servers, PaaS, and Docker
- Dozens of tips, tricks, and techniques
About the Reader
This book assumes you're familiar with Go language basics and the general concepts of web development.
About the Author
Sau Sheong Chang is Managing Director of Digital Technology at Singapore Power and an active contributor to the Ruby and Go communities.
Table of Contents
- PART 1 GO AND WEB APPLICATIONS
- Go and web applications
- Go ChitChat PART 2 BASIC WEB APPLICATIONS
- Handling requests
- Processing requests
- Displaying content
- Storing data PART 3 BEING REAL
- Go web services
- Testing your application
- Leveraging Go concurrency
- Deploying Go
34.99
In Stock
5
1
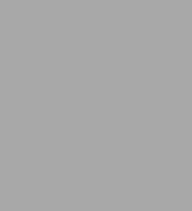
Go Web Programming
312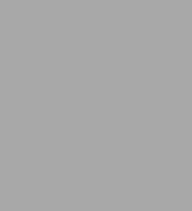
Go Web Programming
312
34.99
In Stock
From the B&N Reads Blog