C++ in 24 Hours, Sams Teach Yourself
Sams Teach Yourself C++ in 24 Hours is a hands-on guide to the C++ programming language. Readers are provided with short, practical examples that illustrate key concepts, syntax, and techniques.
Using a straightforward approach, this fast and friendly tutorial teaches you everything you need to know, from installing and using a compiler, to debugging the programs you’ve created, to what’s new in C++14.
Step-by-step instructions carefully walk you through the most common C++ programming tasks
Quizzes and exercises at the end of each chapter help you test yourself to make sure you’re ready to go on
Learn how to...
1122043150
Using a straightforward approach, this fast and friendly tutorial teaches you everything you need to know, from installing and using a compiler, to debugging the programs you’ve created, to what’s new in C++14.
Step-by-step instructions carefully walk you through the most common C++ programming tasks
Quizzes and exercises at the end of each chapter help you test yourself to make sure you’re ready to go on
Learn how to...
- Install and use a C++ compiler for Windows, Mac OS X, or Linux
- Build object-oriented programs in C++
- Master core C++ concepts such as functions and classes
- Add rich functionality with templates and lambda expressions
- Debug your programs for flawless code
- Learn exception and error-handling techniques
- Put to use the new features in C++14, the latest version of the language
- Create and use templates
- Control program flow with loops
- Store information in arrays and strings
- Declare and use pointers
- Use operator overloading
- Extend classes with inheritance
- Use polymorphism and derived classes
- Employ object-oriented analysis and design
C++ in 24 Hours, Sams Teach Yourself
Sams Teach Yourself C++ in 24 Hours is a hands-on guide to the C++ programming language. Readers are provided with short, practical examples that illustrate key concepts, syntax, and techniques.
Using a straightforward approach, this fast and friendly tutorial teaches you everything you need to know, from installing and using a compiler, to debugging the programs you’ve created, to what’s new in C++14.
Step-by-step instructions carefully walk you through the most common C++ programming tasks
Quizzes and exercises at the end of each chapter help you test yourself to make sure you’re ready to go on
Learn how to...
Using a straightforward approach, this fast and friendly tutorial teaches you everything you need to know, from installing and using a compiler, to debugging the programs you’ve created, to what’s new in C++14.
Step-by-step instructions carefully walk you through the most common C++ programming tasks
Quizzes and exercises at the end of each chapter help you test yourself to make sure you’re ready to go on
Learn how to...
- Install and use a C++ compiler for Windows, Mac OS X, or Linux
- Build object-oriented programs in C++
- Master core C++ concepts such as functions and classes
- Add rich functionality with templates and lambda expressions
- Debug your programs for flawless code
- Learn exception and error-handling techniques
- Put to use the new features in C++14, the latest version of the language
- Create and use templates
- Control program flow with loops
- Store information in arrays and strings
- Declare and use pointers
- Use operator overloading
- Extend classes with inheritance
- Use polymorphism and derived classes
- Employ object-oriented analysis and design
25.49
In Stock
5
1
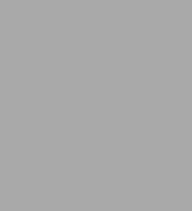
C++ in 24 Hours, Sams Teach Yourself
480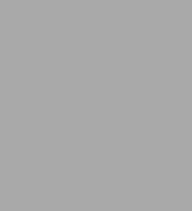
C++ in 24 Hours, Sams Teach Yourself
480
25.49
In Stock
Product Details
ISBN-13: | 9780134192550 |
---|---|
Publisher: | Pearson Education |
Publication date: | 08/02/2016 |
Series: | Sams Teach Yourself |
Sold by: | Barnes & Noble |
Format: | eBook |
Pages: | 480 |
File size: | 19 MB |
Note: | This product may take a few minutes to download. |
Age Range: | 18 Years |
About the Author
From the B&N Reads Blog