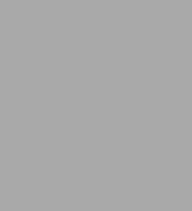
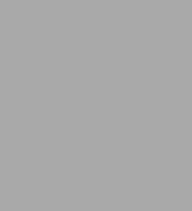
Paperback(1ST)
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
Related collections and offers
Overview
- Detailed coverage of AppleScript Version 1.4 and beyond on Mac OS 9 and Mac OS X.
- Complete descriptions of AppleScript language features, such as data types, flow-control statements, functions, object-oriented features (script objects and libraries), and other syntactical elements.
- Descriptions and hundreds of code samples on programming the various "scriptable" system components, such as the Finder, File Sharing, File Exchange, Network scripting, Web scripting, Apple System Profiler, the ColorSync program, and the numerous powerful language extensions called "osax" or scripting additions. Most other AppleScript books are hopelessly out of date. AppleScript in a Nutshell covers the latest updates and improvements with practical, easy to understand tips, including:
- Using AppleScript as a tool for distributed computing, an exciting development that Apple Computer calls "program linking over IP." Programmers can now do distributed computing with Macs over TCP/IP networks, including controlling remote applications with AppleScript and calling AppleScript methods on code libraries that are located on other machines.
- Using the Sherlock find application to automate web and network searching.
- Insights on scripting new Apple technologies such as Apple Data Detectors, Folder Actions, Keychain Access, and Apple Verifier. AppleScript in a Nutshell is a high-end handbook at a low-end pricean essential desktop reference that puts the full power of this user-friendly programming language into every AppleScript user's hands.
Product Details
ISBN-13: | 9781565928411 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 06/29/2001 |
Series: | In a Nutshell (O'Reilly) |
Edition description: | 1ST |
Pages: | 526 |
Product dimensions: | 6.00(w) x 9.00(h) x 0.99(d) |
About the Author
Read an Excerpt
Chapter 7: Flow-Control Statements
The flow-control statements in AppleScript orchestrate the "flow" or the order in which the code statements execute in your scripts. Programmers will be familiar with AppleScript's if
conditional statements, which are very similar to the syntax of Visual Basic, Perl, and other languages. These statements execute code only if the tested conditions are true
. AppleScript handles loops in script code with several variations of the repeat
statement, similar to the "for," "foreach," or "for each" statements in other languages. The repeat
flow-control construct repeats the execution of code a specified number of times or for each member of a container, such as a list
type. Or, it repeats a code phrase a specified number of times:
repeat 100 times...end repeat
You will be pleased to know that AppleScript has more than adequate error-trapping capabilities. This is accomplished by enclosing the statements that may raise errors in a try...end try
statement block. In addition, you have already seen dozens of examples of the tell..end tell
statement in earlier chapters. These statements specify the objects, usually application objects, that receive the commands or Apple events that your script sends. You specify the targets of different script commands by using these tell
statements.
You can nest flow-control statements within other flow-control statements. Most of these statements end, appropriately, with the reserved word end
, optionally followed by the statement identifier, such as tell
or repeat
. An example is:
tell app "Photoshop 5.5"...end tell
The if
and tell
statements allow "simple" rather than "compound" usage, such as:
if (current date) > date "1/1/2001" then display dialog "Welcome to 2001"
These simple statements appear on one line, they do not contain other code statements, and they do not need to be completed with the end
reserved word. This code shows some nested flow-control statements and simple statements:
tell application "Finder"
set freeMemoryBlock to largest free block
(* Here's a simple statement; no 'end' is necessary *)
if freeMemoryBlock < 10000000 then display dialog ¬
"Memory is getting low"
set listOfProcesses to name of processes
if "BBEdit 5.0" is not in listOfProcesses then (* compound 'if'
statement *)
tell application "BBEdit 5.0" to run -- simple 'tell' statement
end if
end tell
Suffice it to say, flow-control statements are how AppleScript derives much of its power and complexity. You will develop very few scripts that do not use at least one flow-control statement. Table 7-1 lists the statements that this chapter describes.
considering |
repeat with loop variable |
continue |
repeat integer times |
error |
return |
exit |
tell simple statement |
if simple statement |
tell compound statement |
if compound statement |
try |
ignoring |
using terms from |
repeat |
with timeout |
repeat until |
with transaction |
repeat while |
|
considering [but ignoring] end [considering]
Syntax
Considering case
"animal" is equal to "AniMal" -- returns false
end considering
Description
Use considering
statements to specify the elements that should be considered during string comparisons and communications with other applications. The statements that constitute the comparison are enclosed in the considering...end
considering
block. This statement block affects how each of its enclosed statements is processed. The considering
statement can also alter AppleScript's default behavior for the code that is executed prior to the end of the considering
statement (signaled by an end
or end considering
phrase). For example, if you wanted to compare two strings and take upper- or lowercase characters into account, but ignore any white space in the strings, then you would use the statement: considering case but ignoring white space...end
considering
. AppleScript's default behavior is to consider elements such as case, white space, and punctuation when it compares strings for equality. The following constants can also be used in the considering statement (Chapter 6, Variables and Constants, discusses AppleScript's constants):
application responses
case
diacriticals
expansion
hyphens
punctuation
(i.e.,. , ? : ; ! \ ' " `)
white space
AppleScript considers by default an application's responses to any Apple events that your script sends them. You can use the ignoring
statement to ignore responses from an application, as in considering case but ignoring application responses.
There are a few instances when ignoring application responses might make sense, such as when you are sending quit commands to several running processes. If one of the processes responds to the command with an error, then the script ignores its response (as well as any other application response) and thus prevents it from disrupting the execution of the rest of the script. See "ignoring" in this chapter for more details.
Examples
This code shows how to use this statement with a fairly complex string comparison:
tell application "Finder"
considering case but ignoring punctuation, white space and hyphens
set theTruth to ("voracious appetite" is equal to "voracious, ¬
appetite") --returns true
end considering
end tell
The example tells AppleScript which elements to consider and ignore when executing the string comparison within the considering
statement block. Since white space, hyphens, and punctuation should be ignored in the comparison, the two strings turn out the same. Therefore, the theTruth
variable is set to true
. If you are wondering why you would ever ignore these elements in a string comparison, programs often deal with a lot of junk characters and tokens, such as markup-language elements, which are returned from applications or web pages. The considering
statement allows you, in a minimal way, to filter out elements that you do not want to include in string comparisons (unfortunately, you will have to write custom functions or use an HTML-parsing osax to filter out the common < > characters in hypertext markup language [HTML], as AppleScript does not consider them to be "punctuation").
continue
Syntax
On parMethod(int )
If somethingTrue then
(* code statements here *)
else
continue parMethod(int) -- call parent script's parMethod version
end if
end parMethod
Description
The continue
statement is used to call a parent script's method from a child script. In AppleScript, a child script can inherit properties and methods from a parent script. This topic is covered in Chapter 9, Script Objects and Libraries. The child script specifies its parent (if it has one) by declaring a parent property at the top of the script:
property parent : NameOfScript
The NameOfScript part can be either the name of a script object or an application, such as:
application "Finder"
A child script inherits the methods of a parent; it does not have to define these methods. However, the child script can "override" the parent method(s) by redefining them in the body of the script. Within these redefined methods, it can use the continue
statement to call the parent method. The following example constitutes a child script that calls its parent's version of the parMethod function based on the magnitude of the numerical argument passed to the method. The child object handles real
numbers and the parent handles integers.
Examples
On parMethod(int )
If (class of int is real) then
Return (int * 2)
Else
continue parMethod(int) (* it's an integer so call the parent
parMethod *)
end if
end parMethod
error
Syntax
error myErrText number 9000 -- myErrText contains the error description
Description
The error
statement allows you to raise an error based on certain script conditions. Why would you ever want to raise an error? You may want to catch an error in a function but handle the error in the part of the code that called the function, higher up in the call chain. So you would use the error
statement to pass the error up to the calling function, such as in the following example. This is similar to "throwing" an exception in Java.
Examples
This example uses a getNumber method to get a number from the user, but it does not bother (for the sake of demonstration) to check the entry to ensure that the user has entered a valid number. If the user enters data that is not a number then the statement:
return (theNum as number)
causes an error, because AppleScript cannot coerce non-numeric characters to a number
. To be specific, this is AppleScript error number -1700. The getNumber method catches the error and uses an error
statement to pass the original error's error message and number back to the calling handler (in this case the script's run handler), which then catches the "re-raised" error and displays a message:
on run
try
display dialog "Your number is: " & (getNumber( ) as text)
on error errmesg number errn
display dialog errmesg & return & return & "error number: " & ¬
(errn as text)
end try
end run
on getNumber( )
set theNum to (text returned of (display dialog ¬
"Please enter a number:" default answer ""))
try
return (theNum as number)
on error errmesg number errnumber
error errmesg number errnumber
end try
end getNumber
The error
statement also gives the scripter more control on how program errors are handled. For example, you can catch an error in a script with a try
block (see "try" later in this chapter), examine the nature of the error, then re-raise the error with the error
statement providing a more lucid error message to the user. This is best illustrated with the following code, which catches an error caused by coercing a non-numeric string to a real
data type:
(* use the display-dialog scripting addition to ask the user to enter a number *)
set aNum to the text returned of (display dialog "Enter a number" ¬
default answer "")
try
set aNum to aNum as real (* non-numeric string like "10ab" will raise an error *)
on error number errNumber
set myErrText to "Can't coerce the supplied text to a real: " & ¬
return & "The AS error number is " & errNumber
error myErrText number 9000 -- add your own error number
end try
The code first asks the user to enter a number, using the display dialog scripting addition. This produces a dialog box with a text-entry field. If the user enters some text that cannot be coerced to a number
, such as 10ab
(the included letters ab
cause the coercion to fail), the expression:
set aNum to aNum as real
causes the script to raise an error. The try
block catches the error, and then processes the statements following the on error
code. These statements include:
error myErrText number 9000
which produces an AppleScript error-dialog box and adds the scripter's custom message (stored in the variable myErrText
). It also provides a custom error number of 9000. You can create your own groups of error numbers or variables for certain error conditions, which your script can then identify and respond to with more accuracy and clarity than if the scripter only relied on AppleScript's error numbers.
The next two examples illustrate the setup and usage of custom error variables. The first example is a script that contains several user-defined error variables for some common errors that occur in AppleScripts. This script is loaded into the current script using the load script scripting addition (Appendix A, Standard Scripting Additions, discusses scripting additions, or osaxen). The example only contains three constants, but it could define dozens of them to accommodate most or all of the possible script errors that could occur. The constants are set to the actual values that AppleScript assigns to the errors that represent, for example, the failure to coerce data from one type to another (i.e., error number -1700):
set FAILED_COERCION to -1700
set MISSING_PARAMETER to -1701
set TIMEDOUT_APPLEEVENT to -1712
You can then test for certain errors and, if you discover them, display more informative messages or take some other appropriate action. For example, the script in the following code sets the variable objErrors
to the script object defined in the prior example. It then uses the FAILED_COERCION
and TIMEDOUT_APPLEEVENT
constants from that object to test for these error conditions. In other words, the TIMEDOUT_APPLEEVENT
variable contains AppleScript's actual error number for Apple events that time out (-1712), but it is easier to remember if it is stored in a variable with a coherent name. If either of these errors is detected, the error statement is used to produce a dialog box with your own error message:
set objErrors to (load script file "HFSA2gig:nutshell book:demo ¬ scripts:scr_0504")(* this script object contains
the user-defined error variables *)set userReply to the text returned of (display dialog ¬
"Please enter a number" default answer "")
try
set aNum to userReply as real (* if the user doesn't provide a number
this statement will fail and the try block will catch the error *)
on error errM number errNumber
if errNumber is equal to (objErrors's FAILED_COERCION) then
(* FAILED_COERCION is a property of the script object stored in objError *)
error "The number you provided was not a valid integer or real."
else if errNumber is equal to (objErrors's TIMEDOUT_APPLEEVENT) then
error "For some reason AppleScript timed out."
else -- default error message for all other errors
set defMessage to "Sorry, AppleScript error number: " & errNumber & ¬ "occurred in the script. Here's
AppleScript's error description: " & errM error defMessageend if
end try
The error
statement includes a number of optional parameters. It is important to remember that you supply the values for these parameters (if you want to use them). With try
blocks and on error
statements, AppleScript itself will fill these parameters with values (see "try" later in this chapter):
- A non-labeled parameter that contains the text describing the error, as in
error "The Apple event timed out"
. - A labeled parameter that identifies the error number, such as
error "The
Apple event timed out" number 9000
. You use the keywordnumber
followed by theinteger
. If you do not include an error number, AppleScript gives this parameter a default value of -2700. - A labeled parameter that identifies the object that caused the error, as in
error "The Apple event timed out" number 9000 from userReply
. You use the keywordfrom
followed by a reference to the object, in this case the variable that caused the coercion error. - A labeled parameter that involves the reserved words
partial result
followed by a value of typelist
. If the command that caused the error involved the receipt of return values from multiple objects (e.g., a command sent to several database files to get some data), then thelist
value contains any of the values that were successfully received before the error halted the operation. AppleScript gives this parameter a default value of the empty list ({ }). - A
to
keyword or label followed by a word that identifies a class type, such asboolean
,string
, orinteger
. If the command that caused the error received the wrong type of parameter value, then theto
labeled parameter will identify the correct data type that the parameter expected.
The following example demonstrates how to pass the information about an AppleScript error to an error
statement. The script intentionally raises an error by using a string
instead of a boolean
expression in an if
statement. Then it passes the error data as a long string
to an error
statement:
try
if "not a boolean" then (* this causes an error, caught in the try block *)
beep 2
end if
on error errMessage number errNum from errSource partial result ¬
errList to class_constant -- various variables store information about the error
set bigmessage to "The error is: " & errMessage & return & ¬
"The number is: " & errNum & return & "The source is: " & errSource
error bigmessage -- error statement displays dialog box to user
end try
exit [repeat]
Syntax
repeat 2 times
exit repeat
end repeat
Description
The exit
statement causes the flow of script execution to leave the exit
statement's repeat
loop. The execution then resumes with the script code following the repeat
loop. exit
can only be used inside of a repeat
loop, regardless of the repeat
-loop variation. Using exit
is the conventional way to exit a repeat
loop that has no conditional statement associated with it, as shown in this example. In other words, this form of repeat
is an infinite loop:
repeat
set userReply to the text returned of (display dialog "Want to get ¬
out of this endless loop?" default answer "")
if userReply is "yes" then exit repeat
end repeat
This endless loop can also be exited by clicking the Cancel button on the dialog produced by display dialog, which terminates the execution of the script.
if simple statement
Syntax
If theBool is true then exit repeat
Description
AppleScript supports the simple if
statement that is similar to Perl's. You can use a statement such as the following...
Table of Contents
- Preface
- Introduction to AppleScript
- Chapter 1: AppleScript: An Introduction
- Chapter 2: Using Script Editor with OS 9 and OS X
- AppleScript Language Reference
- Chapter 3: Data Types
- Chapter 4: Operators
- Chapter 5: Reference Forms
- Chapter 6: Variables and Constants
- Chapter 7: Flow-Control Statements
- Chapter 8: Subroutines
- Chapter 9: Script Objects and Libraries
- Scripting Mac OS 9 Applications
- Chapter 10: Apple Guide and Help Viewer
- Chapter 11: Apple System Profiler
- Chapter 12: Keychain Scripting and Apple Verifier
- Chapter 13: Desktop Printer Manager
- Chapter 14: Mac OS 9 Finder Commands
- Chapter 15: Mac OS 9 Finder Classes
- Chapter 16: Network Setup Scripting
- Chapter 17: Scripting Sherlock 2
- Chapter 18: URL Access Scripting
- Scripting Mac OS 9 Control Panels and Extensions
- Scripting the Mac OS X System
- Chapter 32: Scripting the OS X Desktop
- Chapter 33: Scripting Mail
- Chapter 34: Executing Scripts with the Terminal App
- Chapter 35: Scripting TextEdit
- Appendixes
- Standard Scripting Additions
- AppleScript Resources
- Colophon