Advanced UNIX Programming / Edition 2 available in Paperback, eBook
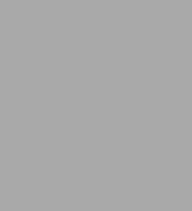
- ISBN-10:
- 0131411543
- ISBN-13:
- 9780131411548
- Pub. Date:
- 04/29/2004
- Publisher:
- Pearson Education
- ISBN-10:
- 0131411543
- ISBN-13:
- 9780131411548
- Pub. Date:
- 04/29/2004
- Publisher:
- Pearson Education
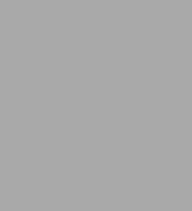
Buy New
$64.99Buy Used
$15.08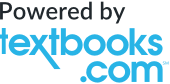
-
SHIP THIS ITEM— This item is available online through Marketplace sellers.
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
This item is available online through Marketplace sellers.
-
SHIP THIS ITEM
Temporarily Out of Stock Online
Please check back later for updated availability.
This item is available online through Marketplace sellers.
Overview
Product Details
ISBN-13: | 9780131411548 |
---|---|
Publisher: | Pearson Education |
Publication date: | 04/29/2004 |
Series: | Addison-Wesley Professional Computing Series |
Edition description: | 2ND |
Pages: | 736 |
Product dimensions: | 7.00(w) x 9.10(h) x 1.60(d) |
About the Author
Table of Contents
Preface.1 Fundamental Concepts.
A Whirlwind Tour of UNIX and Linux. Versions of UNIX. Using System Calls. Error Handling. UNIX Standards. Common Header File. Dates and Times. About the Example Code. Essential Resources.
2. Basic File I/O.
Introduction to File I/O. File Descriptors and Open File Descriptions. Symbols for File Permission Bits. open and creat System Calls. umask System Call. unlink System Call. Creating Temporary Files. File Offsets and O_APPEND. write System Call. read System Call. close System Call. User Buffered I/O. lseek System Call. pread and pwrite System Calls. readv and writev System Calls. Synchronized I/O. truncate and ftruncate System Calls.
3. Advanced File I/O.
Introduction. Disk Special Files and File Systems. Hard and Symbolic Links. Pathnames. Accessing and Displaying File Metadata. Directories. Changing an I-Node. More File-Manipulation Calls. Asynchronous I/O.
4. Terminal I/O.
Introduction. Reading from a Terminal. Sessions and Process Groups (Jobs). ioctl System Call. Setting Terminal Attributes. Additional Terminal-Control System Calls. Terminal-Identification System Calls. Full-Screen Applications. STREAMS I/O. Pseudo Terminals.
5. Processes and Threads.
Introduction. Environment. exec System Calls. Implementing a Shell (Version 1). fork System Call. Implementing a Shell (Version 2). exit System Calls and Process Termination. wait, waitpid, and waitid System Calls. Signals, Termination, and Waiting. Implementing a Shell (Version 3). Getting User and Group Ids. Setting User and Group Ids. Getting Process Ids. chroot System Call. Getting and Setting the Priority. Process Limits. Introduction to Threads. The Blocking Problem.
6. Basic Interprocess Communication.
Introduction. Pipes. dup and dup2 System Calls. A Real Shell. Two-Way Communication with Unidirectional Pipes. Two-Way Communication with Bidirectional Pipes.
7. Advanced Interprocess Communication.
Introduction. FIFOs, or Named Pipes. An Abstract Simple Messaging Interface (SMI). System V IPC (Interprocess Communication). System V Message Queues. POSIX IPC. POSIX Message Queues. About Semaphores. System V Semaphores. POSIX Semaphores. File Locking. About Shared Memory. System V Shared Memory. POSIX Shared Memory. Performance Comparisons.
8. Networking and Sockets.
Socket Basics. Socket Addresses. Socket Options. Simple Socket Interface (SSI). Socket Implementation of SMI. Connectionless Sockets. Out-of-Band Data. Network Database Functions. Miscellaneous System Calls. High-Performance Considerations.
9. Signals and Timers.
Signal Basics. Waiting for a Signal. Miscellaneous Signal System Calls. Deprecated Signal System Calls. Realtime Signals Extension (RTS). Global Jumps. Clocks and Timers.
Appendix A. Process Attributes.
Appendix B. Ux: A C++ Wrapper for Standard UNIX Functions.
Appendix C. Jtux: A Java/Jython Interface to Standard UNIX Functions.
Appendix D. Alphabetical and Categorical Function Lists.
References.
Index.
Preface
Preface
This book updates the 1985 edition of Advanced UNIX Programming to cover a few changes that have occurred in the last eighteen years. Well, maybe 'few' isn't the right word! And 'updates' isn't right either. Indeed, aside from a sentence here and there, this book is all new. The first edition included about 70 system calls; this one includes about 300. And none of the UNIX standards and imple-mentations discussed in this bookPOSIX, Solaris, Linux, FreeBSD, and Darwin (Mac OS X)were even around in 1985. A few sentences from the 1985 Preface, however, are among those that I can leave almost unchanged:The subject of this book is UNIX system callsthe interface between the UNIX kernel and the user programs that run on top of it. Those who interact only with commands, like the shell, text editors, and other application programs, may have little need to know much about system calls, but a thorough knowledge of them is essential for UNIX programmers. System calls are the only way to access kernel facilities such as the file system, the multitasking mechanisms, and the interprocess communication primitives.
System calls define what UNIX is. Everything elsesubroutines and commandsis built on this foundation. While the novelty of many of these higher-level programs has been responsi-ble for much of UNIX's renown, they could as well have been programmed on any modern operating system. When one describes UNIX as elegant, simple, efficient, reliable, and porta-ble, one is referring not to the commands (some of which are none of these things), but to the kernel.That's all still true, except that, regrettably, the programming interface to the ker-nel is no longer elegant or simple. In fact, because UNIX development has splintered into many directions over the last couple of decades, and because the principal standards organization, The Open Group, sweeps up almost everything that's out there (1108 functions altogether), the interface is clumsy, inconsistent, redundant, error-prone, and confusing. But it's still efficient, reliably imple-mented, and portable, and that's why UNIX and UNIX-like systems are so successful. Indeed, the UNIX system-call interface is the only widely imple-mented portable one we have and are likely to have in our lifetime.
To sort things out, it's not enough to have complete documentation, just as the Yellow Pages isn't enough to find a good restaurant or hotel. You need a guide that tells you what's good and bad, not just what exists. That's the purpose of this book, and why it's different from most other UNIX programming books. I tell you not only how to use the system calls, but also which ones to stay away from because they're unnecessary, obsolete, improperly implemented, or just plain poorly designed.
Here's how I decided what to include in this book: I started with the 1108 func-tions defined in Version 3 of the Single UNIX Specification and eliminated about 590 Standard C and other library functions that aren't at the kernel-interface level, about 90 POSIX Threads functions (keeping a dozen of the most important), about 25 accounting and logging functions, about 50 tracing functions, about 15 obscure and obsolete functions, and about 40 functions for scheduling and other things that didn't seem to be generally useful. That left exactly 307 for this book. (See Appendix D for a list.) Not that the 307 are all goodsome of them are use-less, or even dangerous. But those 307 are the ones you need to know.
This book doesn't cover kernel implementation (other than some basics), writing device drivers, C programming (except indirectly), UNIX commands (shell, vi, emacs, etc.), or system administration.
There are nine chapters: Fundamental Concepts, Basic File I/O, Advanced File I/O, Terminal I/O, Processes and Threads, Basic Interprocess Communication, Advanced Interprocess Communication, Networking and Sockets, and Signals and Timers. Read all of Chapter 1, but then feel free to skip around. There are lots of cross-references to keep you from getting lost.
Like the first edition, this new book includes thousands of lines of example code, most of which are from realistic, if simplified, applications such as a shell, a full- screen menu system, a Web server, and a real-time output recorder. The examples are all in C, but I've provided interfaces in Appendices B and C so you can pro-gram in C++, Java, or Jython (a variant of Python) if you like.
The text and example code are just resources; you really learn UNIX program-ming by doing it. To give you something to do, I've included exercises at the end of each chapter. They range in difficulty from questions that can be answered in a few sentences to simple programming problems to semester-long projects.I used four UNIX systems for nuts-and-bolts research and to test the examples: Solaris 8, SuSE Linux 8 (2.4 kernel), FreeBSD 4.6, and Darwin (the Mac OS X kernel) 6.8. I kept the source on the FreeBSD system, mounted on the others with NFS or Samba.
I edited the code with TextPad on a Windows system and accessed the four test systems with Telnet or SSH (PuTTY) or with the X Window System (XFree86 and Cygwin). Having the text editor and the four Telnet/SSH/Xterm windows open on the same screen turned out to be incredibly convenient, because it takes only a few minutes to write some code and check it out on the four systems. In*addition, I usually had one browser window open to the Single UNIX Specifi-cation and one to Google, and another window running Microsoft Word for editing the book. With the exception of Word, which is terrible for big documents like books (crashes, mixed-up styles, weak cross-referencing, flakey document- assembly), all of these tools worked great. I used Perl and Python for various things like extracting code samples and maintaining the database of system calls.