A fully updated tutorial on the basics of the Python programming language for science students
Python is a computer programming language that has gained popularity throughout the sciences. This fully updated second edition of A Student's Guide to Python for Physical Modeling aims to help you, the student, teach yourself enough of the Python programming language to get started with physical modeling. You will learn how to install an open-source Python programming environment and use it to accomplish many common scientific computing tasks: importing, exporting, and visualizing data; numerical analysis; and simulation. No prior programming experience is assumed.
This guide introduces a wide range of useful tools, including:
- Basic Python programming and scripting
- Numerical arrays
- Two- and three-dimensional graphics
- Animation
- Monte Carlo simulations
- Numerical methods, including solving ordinary differential equations
- Image processing
Numerous code samples and exercises—with solutions—illustrate new ideas as they are introduced. This guide also includes supplemental online resources: code samples, data sets, tutorials, and more. This edition includes new material on symbolic calculations with SymPy, an introduction to Python libraries for data science and machine learning (pandas and sklearn), and a primer on Python classes and object-oriented programming. A new appendix also introduces command line tools and version control with Git.
A fully updated tutorial on the basics of the Python programming language for science students
Python is a computer programming language that has gained popularity throughout the sciences. This fully updated second edition of A Student's Guide to Python for Physical Modeling aims to help you, the student, teach yourself enough of the Python programming language to get started with physical modeling. You will learn how to install an open-source Python programming environment and use it to accomplish many common scientific computing tasks: importing, exporting, and visualizing data; numerical analysis; and simulation. No prior programming experience is assumed.
This guide introduces a wide range of useful tools, including:
- Basic Python programming and scripting
- Numerical arrays
- Two- and three-dimensional graphics
- Animation
- Monte Carlo simulations
- Numerical methods, including solving ordinary differential equations
- Image processing
Numerous code samples and exercises—with solutions—illustrate new ideas as they are introduced. This guide also includes supplemental online resources: code samples, data sets, tutorials, and more. This edition includes new material on symbolic calculations with SymPy, an introduction to Python libraries for data science and machine learning (pandas and sklearn), and a primer on Python classes and object-oriented programming. A new appendix also introduces command line tools and version control with Git.
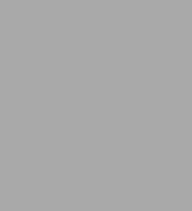
A Student's Guide to Python for Physical Modeling: Second Edition
240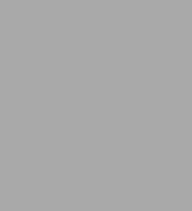
A Student's Guide to Python for Physical Modeling: Second Edition
240eBook
Related collections and offers
Product Details
ISBN-13: | 9780691223667 |
---|---|
Publisher: | Princeton University Press |
Publication date: | 08/03/2021 |
Sold by: | Barnes & Noble |
Format: | eBook |
Pages: | 240 |
Sales rank: | 300,175 |
File size: | 11 MB |
Note: | This product may take a few minutes to download. |